Turn your head and check out this post [How to: Easily rotate the axis labels of a Silverlight/WPF Toolkit chart]
When someone asked me how to rotate the axis labels of a chart from the Data Visualization package of the Silverlight Toolkit/WPF Toolkit earlier today, I realized it was time for a quick blog post. Because when I've answered a question two or three times, it's usually a pretty good sign that I'll keep on answering it for some time. I usually try to head that kind of thing off at the pass, so here's my post on the topic for the benefit of future generations.
The typical scenario here is that someone has a chart and it's working well, but their axis labels are very long and end up overlapping - even after the default axis behavior of putting them in alternating rows to prevent such a problem kicks in:
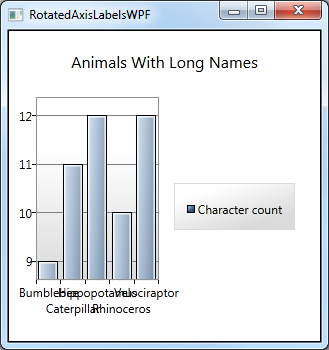
The typical solution is to rotate the axis labels - and it's easy once you know where to look. The key here is to customize the Template
of the AxisLabel
instances that are used to render the labels. And it's quite simple to do so by providing a Style
with a Template
Setter
for the AxisLabelStyle
property of the Axis
subclass in question:
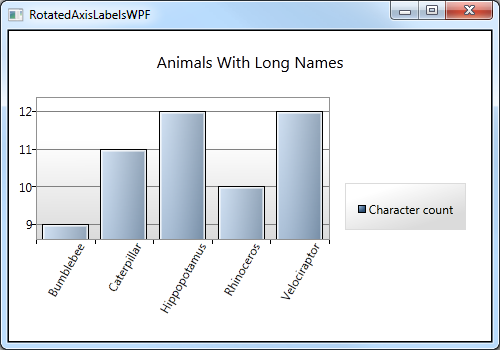
Yeah, it looks great on paper; but that description was a mouthful...
It's probably easier to understand in XAML - here's the complete code for the sample above with the interesting part highlighted:
<Window x:Class="WpfApplication1.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:charting="clr-namespace:System.Windows.Controls.DataVisualization.Charting;assembly=System.Windows.Controls.DataVisualization.Toolkit" xmlns:sys="clr-namespace:System;assembly=mscorlib" xmlns:spec="clr-namespace:System.Collections.Specialized;assembly=System" Title="RotatedAxisLabelsWPF" Width="500" Height="350"> <Grid> <charting:Chart Title="Animals With Long Names"> <charting:ColumnSeries Title="Character count" DependentValueBinding="{Binding Length}" IndependentValueBinding="{Binding}"> <charting:ColumnSeries.ItemsSource> <spec:StringCollection> <sys:String>Bumblebee</sys:String> <sys:String>Caterpillar</sys:String> <sys:String>Hippopotamus</sys:String> <sys:String>Rhinoceros</sys:String> <sys:String>Velociraptor</sys:String> </spec:StringCollection> </charting:ColumnSeries.ItemsSource> <charting:ColumnSeries.IndependentAxis> <charting:CategoryAxis Orientation="X"> <charting:CategoryAxis.AxisLabelStyle> <Style TargetType="charting:AxisLabel"> <Setter Property="Template"> <Setter.Value> <ControlTemplate TargetType="charting:AxisLabel"> <TextBlock Text="{TemplateBinding FormattedContent}"> <TextBlock.LayoutTransform> <RotateTransform Angle="-60"/> </TextBlock.LayoutTransform> </TextBlock> </ControlTemplate> </Setter.Value> </Setter> </Style> </charting:CategoryAxis.AxisLabelStyle> </charting:CategoryAxis> </charting:ColumnSeries.IndependentAxis> </charting:ColumnSeries> </charting:Chart> </Grid> </Window>
Like I said, it's all pretty standard stuff once you know where to look. Of course, you can rotate the labels all the way to 90 degrees if you want them to take the least amount of space possible. But 60 degrees seemed like a suitably rakish angle.
Unfortunately, we can't declare "Mission Accomplished" quite yet... While the Data Visualization assembly itself works exactly the same on WPF and Silverlight, the platforms themselves aren't identical quite yet. Specifically, there's no support for LayoutTransform in Silverlight (and RenderTransform is simply not appropriate here). Fortunately, I've filled the LayoutTransform gap with my LayoutTransformer class - and it's already part of the Silverlight Toolkit!
The syntax changes just a bit, but the concept is exactly the same:
<UserControl x:Class="SilverlightApplication1.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:charting="clr-namespace:System.Windows.Controls.DataVisualization.Charting;assembly=System.Windows.Controls.DataVisualization.Toolkit" xmlns:sys="clr-namespace:System;assembly=mscorlib" xmlns:toolkit="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Toolkit" xmlns:layout="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Layout.Toolkit"> <Grid> <charting:Chart Title="Animals With Long Names"> <charting:ColumnSeries Title="Character count" DependentValueBinding="{Binding Length}" IndependentValueBinding="{Binding}"> <charting:ColumnSeries.ItemsSource> <toolkit:ObjectCollection> <sys:String>Bumblebee</sys:String> <sys:String>Caterpillar</sys:String> <sys:String>Hippopotamus</sys:String> <sys:String>Rhinoceros</sys:String> <sys:String>Velociraptor</sys:String> </toolkit:ObjectCollection> </charting:ColumnSeries.ItemsSource> <charting:ColumnSeries.IndependentAxis> <charting:CategoryAxis Orientation="X"> <charting:CategoryAxis.AxisLabelStyle> <Style TargetType="charting:AxisLabel"> <Setter Property="Template"> <Setter.Value> <ControlTemplate TargetType="charting:AxisLabel"> <layout:LayoutTransformer> <layout:LayoutTransformer.LayoutTransform> <RotateTransform Angle="-60"/> </layout:LayoutTransformer.LayoutTransform> <TextBlock Text="{TemplateBinding FormattedContent}"/> </layout:LayoutTransformer> </ControlTemplate> </Setter.Value> </Setter> </Style> </charting:CategoryAxis.AxisLabelStyle> </charting:CategoryAxis> </charting:ColumnSeries.IndependentAxis> </charting:ColumnSeries> </charting:Chart> </Grid> </UserControl>
Mission accomplished:
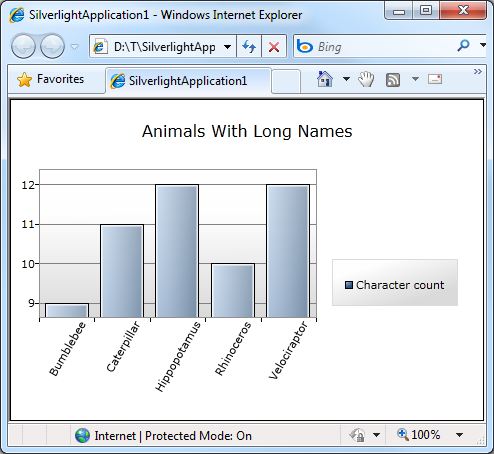
There you have it: AxisLabelStyle
is your new best friend. A friend with benefits, one might say, because there are other cool things you can do by customizing the AxisLabel Style.
So please: go forth and enjoy your new friend!