As the platform evolves, so do the workarounds [Better SetterValueBindingHelper makes Silverlight Setters better-er!]
Back in May, I mentioned that Silverlight 2 and 3 don't support putting a Binding in the Value of a Setter. I explained why this is useful (ex: MVVM, TreeView
expansion, developer/designer separation, etc.) and shared a helper class I wrote to implement the intended functionality on Silverlight. My workaround supported setters for normal DependencyPropertys as well as attached ones, so it covered all the bases. It worked well on both flavors of Silverlight and a bunch of you went off and used SetterValueBindingHelper
successfully in your own projects.
The sun was shining, birds were chirping, and all was right with (that part of) the world...
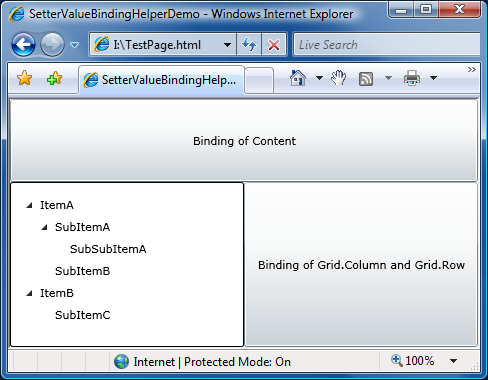
Now flash forward to a few days ago when I was contacted by fellow Silverlight team members RJ Boeke and Vinoo Cherian with a report that certain uses of SetterValueBindingHelper
which worked fine on Silverlight 2 and 3 were likely to break if used in a possible future version of Silverlight that was more consistent with WPF's handling of such things. You can imagine my astonishment and dismay...
Important aside: The Silverlight team takes backward compatibility very seriously, so running any Silverlight 2 or 3 application with SetterValueBindingHelper
on such a future version of Silverlight would continue to work in the expected manner. The Silverlight team makes a concerted effort to ensure that each version of Silverlight is "bug compatible" with previous versions to prevent existing applications from suddenly breaking when a new version of Silverlight comes out. However, were someone to recompile such an application to target a newer release of Silverlight, that application would no longer be subject to the backwards compatibility quirks and would begin seeing the new (more correct/consistent) platform behavior.
RJ and Vinoo pointed out that a more WPF-consistent handling of Style
s would break one of the samples that was part of my original blog post. Specifically, the following example would not have the first Binding
applied (note: per convention, code in italics is wrong):
<Style TargetType="Button"> <!-- WPF syntax: <Setter Property="Grid.Column" Value="{Binding}"/> <Setter Property="Grid.Row" Value="{Binding}"/> --> <Setter Property="local:SetterValueBindingHelper.PropertyBinding"> <Setter.Value> <local:SetterValueBindingHelper Type="System.Windows.Controls.Grid, System.Windows, Version=2.0.5.0, Culture=neutral, PublicKeyToken=7cec85d7bea7798e" Property="Column" Binding="{Binding}"/> </Setter.Value> </Setter> <Setter Property="local:SetterValueBindingHelper.PropertyBinding"> <Setter.Value> <local:SetterValueBindingHelper Type="Grid" Property="Row" Binding="{Binding}"/> </Setter.Value> </Setter> </Style>
What's important to note is that two Setter
s are both setting the same Property
(local:SetterValueBindingHelper.PropertyBinding
) and WPF optimizes this scenario to only apply the last Value
it sees. Clearly, it was time to think about how tweak SetterValueBindingHelper
so it would work with this theoretical future release of Silverlight...
Tangential aside: This kind of platform change wouldn't affect justSetterValueBindingHelper
- any place where multipleSetter
s targeted the sameProperty
would behave differently. But that difference won't matter 99% of the time -SetterValueBindingHelper
is fairly unique in its need that everyValue
be applied.
One idea for a fix is to expose something like PropertyBinding2
from SetterValueBindingHelper
and treat it just like another PropertyBinding
. While that would definitely work, how do we know that two properties is enough? What if you need three or four? No, despite its simplicity, this is not the flexible solution we're looking for.
Taking a step back, what we really want is to somehow provide an arbitrary number of Property
/Binding
pairs instead of being limited to just one. And if you read that last sentence and thought "Collection!", I like the way you think. SetterValueBindingHelper
class we're already using to provide the attached DependencyProperty
and the data for it were also capable of storing a collection of other SetterValueBindingHelper
objects? Yeah, sure, that would work!
So let's lay a few ground rules to help guide us:
- Every current use of
SetterValueBindingHelper
should continue to be valid after we make our changes. In other words, upgrading should be a simple matter of dropping in the newSetterValueBindingHelper.cs
file and that's all. - The new
SetterValueBindingHelper
syntax should work correctly for the current Silverlight 3 release as well as this mythical future version of Silverlight with the WPF-consistentStyle
changes. - The new collection syntax should be easy to use and easy to understand.
- Arbitrary nesting is unnecessary; either someone's using a
SetterValueBindingHelper
on its own, or else they're using it as a container for a single, nested layer ofSetterValueBindingHelper
children. - We could try to be fancy and let children inherit things from their parent, but it's not actually as useful as it seems. Let's not go there and instead keep everything simple and consistent.
Keeping these guidelines in mind, the resulting changes to SetterValueBindingHelper
give us the following alternate representation of the above XAML which works fine on Silverlight 3 today and will also give the desired effect on a possible future version of Silverlight with the WPF optimization:
<Style TargetType="Button"> <!-- WPF syntax: <Setter Property="Grid.Column" Value="{Binding}"/> <Setter Property="Grid.Row" Value="{Binding}"/> --> <Setter Property="delay:SetterValueBindingHelper.PropertyBinding"> <Setter.Value> <delay:SetterValueBindingHelper> <delay:SetterValueBindingHelper Type="System.Windows.Controls.Grid, System.Windows, Version=2.0.5.0, Culture=neutral, PublicKeyToken=7cec85d7bea7798e" Property="Column" Binding="{Binding}"/> <delay:SetterValueBindingHelper Type="Grid" Property="Row" Binding="{Binding}"/> </delay:SetterValueBindingHelper> </Setter.Value> </Setter> </Style>
Aside: The two different ways of identifying Grid above are part of the original sample showing that both ways work - in practice, both instances would use the simple "Grid" form.
Other than the namespace change to "delay" (for consistency with my other samples), the only change here is the extra SetterValueBindingHelper
wrapper you see highlighted. Everything else is pretty much the same and now it works on imaginary versions of Silverlight, too! SetterValueBindingHelper
, please use this latest version; you can rest assured that you're future-proof.
Here's the updated code in its entirety. Please note that I have used a normal (i.e., non-observable) collection, so dynamic updates to the Values
property are not supported. This was a deliberate decision to minimize complexity. (And besides, I've never heard of anyone modifying the contents of a Style
dynamically.)
/// <summary> /// Class that implements a workaround for a Silverlight XAML parser /// limitation that prevents the following syntax from working: /// <Setter Property="IsSelected" Value="{Binding IsSelected}"/> /// </summary> [ContentProperty("Values")] public class SetterValueBindingHelper { /// <summary> /// Optional type parameter used to specify the type of an attached /// DependencyProperty as an assembly-qualified name, full name, or /// short name. /// </summary> [SuppressMessage("Microsoft.Naming", "CA1721:PropertyNamesShouldNotMatchGetMethods", Justification = "Unambiguous in XAML.")] public string Type { get; set; } /// <summary> /// Property name for the normal/attached DependencyProperty on which /// to set the Binding. /// </summary> public string Property { get; set; } /// <summary> /// Binding to set on the specified property. /// </summary> public Binding Binding { get; set; } /// <summary> /// Collection of SetterValueBindingHelper instances to apply to the /// target element. /// </summary> /// <remarks> /// Used when multiple Bindings need to be applied to the same element. /// </remarks> public Collection<SetterValueBindingHelper> Values { get { // Defer creating collection until needed if (null == _values) { _values = new Collection<SetterValueBindingHelper>(); } return _values; } } private Collection<SetterValueBindingHelper> _values; /// <summary> /// Gets the value of the PropertyBinding attached DependencyProperty. /// </summary> /// <param name="element">Element for which to get the property.</param> /// <returns>Value of PropertyBinding attached DependencyProperty.</returns> [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "SetBinding is only available on FrameworkElement.")] public static SetterValueBindingHelper GetPropertyBinding(FrameworkElement element) { if (null == element) { throw new ArgumentNullException("element"); } return (SetterValueBindingHelper)element.GetValue(PropertyBindingProperty); } /// <summary> /// Sets the value of the PropertyBinding attached DependencyProperty. /// </summary> /// <param name="element">Element on which to set the property.</param> /// <param name="value">Value forPropertyBinding attached DependencyProperty.</param> [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "SetBinding is only available on FrameworkElement.")] public static void SetPropertyBinding(FrameworkElement element, SetterValueBindingHelper value) { if (null == element) { throw new ArgumentNullException("element"); } element.SetValue(PropertyBindingProperty, value); } /// <summary> /// PropertyBinding attached DependencyProperty. /// </summary> public static readonly DependencyProperty PropertyBindingProperty = DependencyProperty.RegisterAttached( "PropertyBinding", typeof(SetterValueBindingHelper), typeof(SetterValueBindingHelper), new PropertyMetadata(null, OnPropertyBindingPropertyChanged)); /// <summary> /// Change handler for the PropertyBinding attached DependencyProperty. /// </summary> /// <param name="d">Object on which the property was changed.</param> /// <param name="e">Property change arguments.</param> private static void OnPropertyBindingPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // Get/validate parameters var element = (FrameworkElement)d; var item = (SetterValueBindingHelper)(e.NewValue); if ((null == item.Values) || (0 == item.Values.Count)) { // No children; apply the relevant binding ApplyBinding(element, item); } else { // Apply the bindings of each child foreach (var child in item.Values) { if ((null != item.Property) || (null != item.Binding)) { throw new ArgumentException( "A SetterValueBindingHelper with Values may not have its Property or Binding set."); } if (0 != child.Values.Count) { throw new ArgumentException( "Values of a SetterValueBindingHelper may not have Values themselves."); } ApplyBinding(element, child); } } } /// <summary> /// Applies the Binding represented by the SetterValueBindingHelper. /// </summary> /// <param name="element">Element to apply the Binding to.</param> /// <param name="item">SetterValueBindingHelper representing the Binding.</param> private static void ApplyBinding(FrameworkElement element, SetterValueBindingHelper item) { if ((null == item.Property) || (null == item.Binding)) { throw new ArgumentException( "SetterValueBindingHelper's Property and Binding must both be set to non-null values."); } // Get the type on which to set the Binding Type type = null; if (null == item.Type) { // No type specified; setting for the specified element type = element.GetType(); } else { // Try to get the type from the type system type = System.Type.GetType(item.Type); if (null == type) { // Search for the type in the list of assemblies foreach (var assembly in AssembliesToSearch) { // Match on short or full name type = assembly.GetTypes() .Where(t => (t.FullName == item.Type) || (t.Name == item.Type)) .FirstOrDefault(); if (null != type) { // Found; done searching break; } } if (null == type) { // Unable to find the requested type anywhere throw new ArgumentException(string.Format(CultureInfo.CurrentCulture, "Unable to access type \"{0}\". Try using an assembly qualified type name.", item.Type)); } } } // Get the DependencyProperty for which to set the Binding DependencyProperty property = null; var field = type.GetField(item.Property + "Property", BindingFlags.FlattenHierarchy | BindingFlags.Public | BindingFlags.Static); if (null != field) { property = field.GetValue(null) as DependencyProperty; } if (null == property) { // Unable to find the requsted property throw new ArgumentException(string.Format(CultureInfo.CurrentCulture, "Unable to access DependencyProperty \"{0}\" on type \"{1}\".", item.Property, type.Name)); } // Set the specified Binding on the specified property element.SetBinding(property, item.Binding); } /// <summary> /// Returns a stream of assemblies to search for the provided type name. /// </summary> private static IEnumerable<Assembly> AssembliesToSearch { get { // Start with the System.Windows assembly (home of all core controls) yield return typeof(Control).Assembly; // Fall back by trying each of the assemblies in the Deployment's Parts list foreach (var part in Deployment.Current.Parts) { var streamResourceInfo = Application.GetResourceStream( new Uri(part.Source, UriKind.Relative)); using (var stream = streamResourceInfo.Stream) { yield return part.Load(stream); } } } } }