Dynamic content made easy *redux* [How to: Use the new dynamic population support for Toolkit controls]
I previously blogged a sample demonstrating how to use the AJAX Control Toolkit's dynamic population functionality with a data-bound ModalPopup. That sample was well received, but some of the changes that happened as part of the ASP.NET AJAX Beta 1 and Beta 2 releases have rendered the sample code I originally posted un-runnable on current builds.
No worries - it's a simple matter to update the sample and the exercise should serve as a good example of some of the things that need to be done as part of an upgrade. (For a far more in depth guide to upgrading, please refer to Shawn's AJAX Control Toolkit Migration Guide and follow-up about upgrading Web Services/Methods.)
Referring to the sample code I posted earlier as a starting point, the 5 things we need to do to update it are:
- Change the tag prefix for ScriptManager from "atlas" to "asp" to point to the new location for ScriptManager
- Change the @Register tag to refer to the AjaxControlToolkit by its new name ("AtlasControlToolkit"->"AjaxControlToolkit" twice and "atlasToolkit"->"ajaxToolkit" once)
- Change the corresponding tag prefix for ModalPopupExtender from "atlasToolkit" to "ajaxToolkit"
- Copy the properties of the ModalPopupProperties element to the ModalPopupExtender element and remove the empty ModalPopupProperties element now that the XxxProperties elements are no longer used
- Update the web method definition by making the GetContent method static and adding the [Microsoft.Web.Script.Services.ScriptMethod] attribute
That's it! As you can see, it's mostly simple "find-and-replace" naming changes that can easily be applied to an entire site at once. The XxxProperties change is slightly more involved, but requires very little effort since it's basically just a "cut-and-paste" operation. The web method changes are also a little bit of effort, but the changes themselves are simple and it's easy to find where to make them by searching for the pre-existing "WebMethod" attribute. Nothing here is risky or error-prone and - with the exception of the web method changes - the compiler will report all of them as errors until they're fixed correctly.
For completeness, here's a reminder of what dynamic population looks like in action:
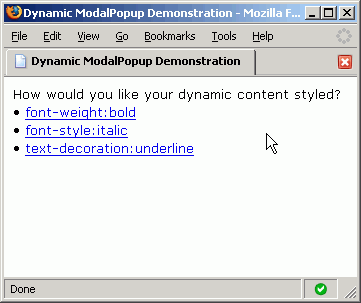
And here's the complete sample code after the changes discussed above have been applied. This sample runs fine on the ASP.NET AJAX Beta 2 and the AJAX Control Toolkit 61106 release:
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit"
TagPrefix="ajaxToolkit" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
[System.Web.Services.WebMethod]
[Microsoft.Web.Script.Services.ScriptMethod]
public static string GetContent(string contextKey)
{
// Create a random color
string color = (new Random()).Next(0xffffff).ToString("x6");
// Use the style specified by the page author
string style = contextKey;
// Display the current time
string time = DateTime.Now.ToLongTimeString();
// Compose the content to return
return "<span style='color:#" + color + "; " + style + "'>" + time + "</span> ";
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>Dynamic ModalPopup Demonstration</title>
<%-- Style the page so it looks pretty --%>
<style type="text/css">
body { font-family:Verdana; font-size:10pt; }
.background { background-color:Gray; }
.popup { width:200px; padding:10px; background-color:White;
border-style:solid; border-color:Black; border-width:2px;
vertical-align: middle; text-align:center; }
</style>
</head>
<body>
<form id="form1" runat="server">
<%-- Atlas pages need a ScriptManager --%>
<asp:ScriptManager ID="ScriptManager1" runat="server" />
<%-- A very simple data source to drive the demonstration --%>
<asp:XmlDataSource ID="XmlDataSource1" runat="server">
<Data>
<items>
<item style="font-weight:bold" />
<item style="font-style:italic" />
<item style="text-decoration:underline" />
</items>
</Data>
</asp:XmlDataSource>
<%-- A simple list of all the data items available --%>
<asp:DataList ID="DataList1" runat="server" DataSourceID="XmlDataSource1">
<HeaderTemplate>
How would you like your dynamic content styled?
</HeaderTemplate>
<ItemTemplate>
• <asp:LinkButton ID="LinkButton" runat="server" Text='<%# Eval("style") %>' />
<%-- The ModalPopupExtender, popping up Panel1 and dynamically populating Panel2 --%>
<ajaxToolkit:ModalPopupExtender ID="ModalPopupExtender" runat="server"
TargetControlID="LinkButton" PopupControlID="Panel1" OkControlID="Button1"
BackgroundCssClass="background" DynamicControlID="Panel2"
DynamicContextKey='<%# Eval("style") %>' DynamicServiceMethod="GetContent" />
</ItemTemplate>
</asp:DataList>
<%-- All ModalPopups share the same popup --%>
<asp:Panel ID="Panel1" runat="server" CssClass="popup" style="display:none;">
<p>This popup popped at <asp:Label ID="Panel2" runat="server" /> and all was well.</p>
<p><asp:Button ID="Button1" runat="server" Text="OK" /></p>
</asp:Panel>
</form>
</body>
</html>
Enjoy!