Your phone can turn into a robot [LayoutTransformer works great on the Windows Phone platform]
The WPF platform offers RenderTransform and LayoutTransform. Silverlight - being considerably smaller and a bit simpler - has only RenderTransform
. Which is usually enough - except when it's not!
So I wrote LayoutTransformControl
a while back in order to bring LayoutTransform
to the Silverlight platform. It was so useful/popular that we included it in the Silverlight Toolkit with the name LayoutTransformer [insert robot sounds here]. That post includes links to a bunch of my posts about LayoutTransformControl
/LayoutTransformer
, but the most important one for newcomers is probably the original "motivational" post.
Here's the relevant portion:
People who want to rotate visual elements in Silverlight are likely to use RotateTransform within RenderTransform - but they may not always get the results they expect! For example, using RenderTransform to achieve the following effect:
![]()
Actually renders like this:
![]()
But the problem isn't with RenderTransform - it's with using the wrong tool for the job! By design, RenderTransform applies its transformations (a rotation in this case) after the layout system has performed its measure/arrange pass. So when the elements in the example are being measured and arranged, the text is still horizontal. It's only after everything has been positioned that the text is finally rotated - and ends up in the "wrong" place. While it's possible to correct for this discrepancy by hard-coding all the relevant offsets in the XAML (very brittle and error-prone) or by adjusting all the offsets in code (only slightly more flexible - and a lot more work), these aren't great alternatives.
The right tool for the job is LayoutTransform which applies its transformations before the layout pass. With LayoutTransform, the text in the example is already rotated by the time the elements are measured and arranged, and the desired effect can be achieved quite simply.
But there's a catch: LayoutTransform doesn't exist in Silverlight [and therefore Windows Phone]...
However, there's no reason to let that stop us. Rotation is rotation whenever it happens, so maybe there's a way to get the already-optimized RenderTransform implementation to do the real work earlier in the layout pass...
Lately, I've been contacted by a number of customers asking if LayoutTransform
worked in Windows Phone applications or having trouble referencing it from the relevant Silverlight 3 Toolkit assembly. So I figured it would be good to verify this for myself and make it even easier for people to use!
There are lots of compelling scenarios for LayoutTransform
, but the most common is definitely rotating text and images. So here's my simple "bookshelf" sample which highlights a few of my favorite programming books:
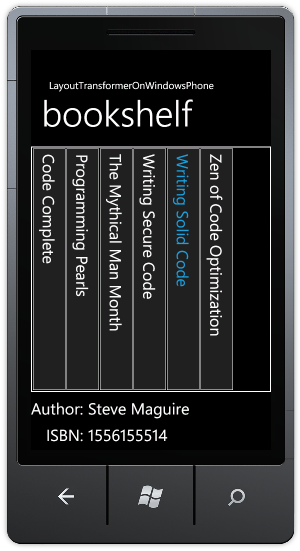
The tricky part of creating a layout like this without LayoutTransformer
would have been getting those vertical book titles aligned properly on the spines of those books without hardcoding a bunch of positioning data (which would have broken as soon as anyone touched the XAML). But LayoutTransformer
makes this a piece of cake - just wrap the content, rotate it, and everything automatically works just how you'd expect it to! You can change font sizes, margins, alignment - whatever - and never have to worry about your layout going funky.
When you're ready to add LayoutTransformer
to your own application, just add a project reference to the LayoutTransformer.dll assembly (found in the root of the ZIP download) and add the appropriate XMLNS to the top of your XAML:
xmlns:toolkit="clr-namespace:System.Windows.Controls;assembly=LayoutTransformer"
After that, wrap anything you want in a LayoutTransformer
and apply the relevant transforms. Here's what the sample uses:
<toolkit:LayoutTransformer> <toolkit:LayoutTransformer.LayoutTransform> <RotateTransform Angle="90"/> </toolkit:LayoutTransformer.LayoutTransform> <Border BorderBrush="{StaticResource PhoneForegroundBrush}" BorderThickness="1"> <TextBlock Text="{Binding Title}" Margin="10"/> </Border> </toolkit:LayoutTransformer>
Yep, it's really that easy!
PS - There's a bug in the default templates of the Windows Phone Developer Tools Beta that makes the books show up with different heights. That problem has been fixed in more recent Tools releases (as demonstrated by the screen capture above), so don't worry when you see that on the Beta - it has nothing to do with LayoutTransformer
.